INTRODUCTION:
Are you new to the world of web development and eager to learn the basics of JavaScript? Look no further! In this comprehensive guide, we’ll take you by the hand and walk you through the process of learning JavaScript from scratch.
JavaScript is a high-level, dynamic, and interpreted programming language primarily used for client-side scripting on the web. It has become one of the most popular programming languages due to its versatility and ability to create interactive web applications. Let’s explore what JavaScript is, its key features, and its various applications.
What is JavaScript?
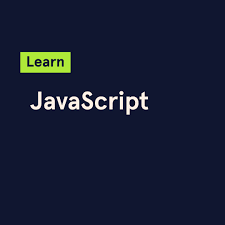
JavaScript (often abbreviated as JS) is a programming language that enables developers to implement complex features on web pages. It allows for the creation of dynamic content, control multimedia, animate images, and much more. Unlike HTML and CSS, which are used for structure and styling, JavaScript adds interactivity to websites.
Key Features of JavaScript
- Dynamic Typing:
- JavaScript uses dynamic typing, meaning that variable types are determined at runtime. This allows developers to assign different data types to the same variable without declaring its type explicitly.
- Example:
javascript let variable = "Hello"; // string variable = 42; // now it's a number
- Interpreted Language:
- JavaScript is an interpreted language, which means it executes code line by line in the browser without the need for a separate compilation step. This makes it easy to test and debug code quickly.
- Event Handling:
- JavaScript can respond to user events like clicks, mouse movements, and keyboard inputs. This feature allows developers to create interactive experiences.
- Example:
javascript document.getElementById("myButton").onclick = function() { alert("Button clicked!"); };
- Object-Oriented Programming:
- JavaScript supports object-oriented programming (OOP) principles, allowing developers to create objects that can encapsulate data and functionality.
- Example:
javascript function Car(make, model) { this.make = make; this.model = model; this.displayInfo = function() { return `${this.make} ${this.model}`; }; } const myCar = new Car("Toyota", "Corolla"); console.log(myCar.displayInfo()); // Outputs: Toyota Corolla
- Cross-Platform Compatibility:
- JavaScript runs on all modern web browsers, making it platform-independent. This means you can write your code once and have it work on any device or operating system.
- Rich Ecosystem:
- There are numerous libraries and frameworks built on top of JavaScript (like React, Angular, and Vue.js) that simplify development and enhance functionality.
- Asynchronous Programming:
- JavaScript supports asynchronous programming through callbacks, promises, and async/await syntax, allowing for non-blocking operations such as fetching data from APIs without freezing the user interface.
Want To Be Become An Expert in HTLM and CSS:
2.Unlock The Secrets Of CSS: A Beginner’s Guide To Learning CSS From Scratch.
Applications of JavaScript
- Web Development: JavaScript is essential for creating interactive websites. It is used alongside HTML and CSS to enhance user experience.
- Mobile App Development: Frameworks like React Native allow developers to build mobile applications using JavaScript.
- Game Development: Many web-based games are created using JavaScript due to its ability to handle graphics and animations.
- Server-Side Development: With environments like Node.js, JavaScript can be used on the server side to handle requests and manage databases.
JavaScript is a versatile language that has transformed web development by enabling interactive and dynamic content on websites. Its features like dynamic typing, event handling, and support for OOP make it a powerful tool for developers. Whether you are building a simple webpage or a complex application, understanding JavaScript is essential in today’s tech landscape. Its wide range of applications—from client-side scripting to server-side development—highlights its importance in modern software development.
Why Learn JavaScript?
JavaScript is an essential skill for any web developer, and learning it can open up a world of creative possibilities. With JavaScript, you can:
– Create interactive web pages that respond to user input
– Develop desktop and mobile applications
– Create games and animations
– Work with popular frameworks like React, Angular, and Vue.js
Basic JavaScript Concepts:
Before diving into the intricacies of JavaScript, it’s essential to grasp some fundamental concepts that form the backbone of the language. Here’s an overview of basic JavaScript concepts, including variables, data types, functions, and conditional statements, along with a brief look at JavaScript syntax.
1. Variables:
**Variables** are used to store and manipulate data in JavaScript. You can declare a variable using three keywords: `let`, `const`, or `var`.
– **`let`:** Allows you to declare variables that can be reassigned later.
“`javascript
let message = “Hello, World!”;
message = “Hello, JavaScript!”; // Reassignment is allowed
“`
– **`const`:** Used to declare variables that cannot be reassigned. It’s generally used for constants.
“`javascript
const pi = 3.14; // This value cannot be changed
“`
– **`var`:** An older way to declare variables. It has function scope and can lead to hoisting issues, so it’s less commonly used in modern JavaScript.
“`javascript
var isLearning = true;
“`
2. Data Types:
JavaScript has several **data types**, which can be categorized into two groups: primitive and reference types.
– **Primitive Data Types:**
– **String:** A sequence of characters.
“`javascript
let name = “Alice”;
“`
– **Number:** Represents both integer and floating-point numbers.
“`javascript
let age = 30;
“`
– **Boolean:** Represents true or false values.
“`javascript
let isStudent = false;
“`
– **Null:** Represents an intentional absence of any value.
“`javascript
let emptyValue = null;
“`
– **Undefined:** Indicates a variable that has been declared but not assigned a value.
“`javascript
let notAssigned;
“`
– **Reference Data Types:**
– **Object:** A collection of key-value pairs.
“`javascript
let person = {
firstName: “John”,
lastName: “Doe”
};
“`
– **Array:** An ordered collection of values.
“`javascript
let fruits = [“Apple”, “Banana”, “Cherry”];
“`
3. Functions:
**Functions** are reusable blocks of code that can take inputs (arguments) and return outputs (values). They help encapsulate logic for better organization and reuse.
– **Function Declaration:**
“`javascript
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet(“Alice”)); // Output: Hello, Alice!
“`
– **Arrow Function:**
“`javascript
const greet = (name) => `Hello, ${name}!`;
console.log(greet(“Bob”)); // Output: Hello, Bob!
“`
4. Conditional Statements:
**Conditional statements** allow you to execute different code based on certain conditions. Common statements include `if`, `else`, and `switch`.
– **If-Else Statement:**
“`javascript
let temperature = 25;
if (temperature > 30) {
console.log(“It’s hot outside!”);
} else {
console.log(“It’s not too hot today.”);
}
“`
– **Switch Statement:**
“`javascript
let day = “Monday”;
switch (day) {
case “Monday”:
console.log(“Start of the week!”);
break;
case “Friday”:
console.log(“Almost weekend!”);
break;
default:
console.log(“Just another day.”);
break;
}
“`
JavaScript Syntax:
JavaScript syntax is similar to other programming languages but has its own unique rules:
– **Indentation:** Use spaces or tabs to indent your code for readability.
– **Comments:** Use `//` for single-line comments and `/* */` for multi-line comments.
“`javascript
// This is a single-line comment
/*
This is a
multi-line comment
*/
“`
– **Variable Declaration:** Use `let`, `const`, or `var` to declare variables.
– **Function Declaration:** Use the `function` keyword to define functions.
Understanding these basic JavaScript concepts—variables, data types, functions, and conditional statements—is crucial for anyone looking to work with the language effectively. These foundational elements will help you write more complex code and build interactive web applications as you progress in your learning journey. By mastering these concepts, you’ll be well-equipped to tackle more advanced topics in JavaScript.
Practical Examples:
Example 1: Print “Hello, World!” to the Console
In this example, we will create a simple JavaScript program that prints “Hello, World!” to the console. This is often the first program written when learning a new programming language.
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Hello World Example</title>
</head>
<body>
<script>
console.log(“Hello, World!”); // This prints “Hello, World!” to the console
</script>
</body>
</html>
“`
Example 2: Create a Function That Returns a Greeting Message:
In this example, we will create a JavaScript function that takes a name as an argument and returns a greeting message. We will then call this function and print the result to the console.
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Greeting Example</title>
</head>
<body>
<script>
function greet(name) {
return `Hello, ${name}!`; // Returns a greeting message
}
console.log(greet(“John”)); // Outputs: Hello, John!
</script>
</body>
</html>
“`
Example 3: Determine Whether a Number is Even or Odd
In this example, we will create a JavaScript program that uses conditional statements to determine whether a number is even or odd. The program checks the number and prints the result to the console.
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Even or Odd Example</title>
</head>
<body>
<script>
let num = 5; // You can change this number to test other values
if (num % 2 === 0) {
console.log(`${num} is even.`); // Outputs if the number is even
} else {
console.log(`${num} is odd.`); // Outputs if the number is odd
}
</script>
</body>
</html>
“`
Summary of Examples:
– **Example 1** demonstrates how to print “Hello, World!” to the console using `console.log()`. This simple command is often used for debugging and testing.
– **Example 2** showcases how to create a function named `greet` that takes an argument (`name`) and returns a personalized greeting message. This illustrates how functions can encapsulate logic for reuse.
– **Example 3** illustrates how to use conditional statements (`if` and `else`) to determine if a number is even or odd based on its modulus with `2`. This example highlights decision-making in programming.
These practical examples provide a hands-on approach to understanding JavaScript’s core functionalities. By experimenting with these snippets, you can deepen your knowledge and gain confidence in using JavaScript for various tasks.
Conclusion:
Learning JavaScript from scratch can seem daunting, but with practice and patience, you can master the basics of JavaScript. Remember to start with simple examples and gradually build up to more complex programs. With JavaScript, the possibilities are endless, and we hope this guide has inspired you to start creating your own amazing web applications.
Disclosure/Disclaimer:
This article contains affiliate links, supporting our educational and informative contents creation.
We appreciate supporting us to create more value and fascinating contents here on our site for educational purposes only.