INTRODUCTION:
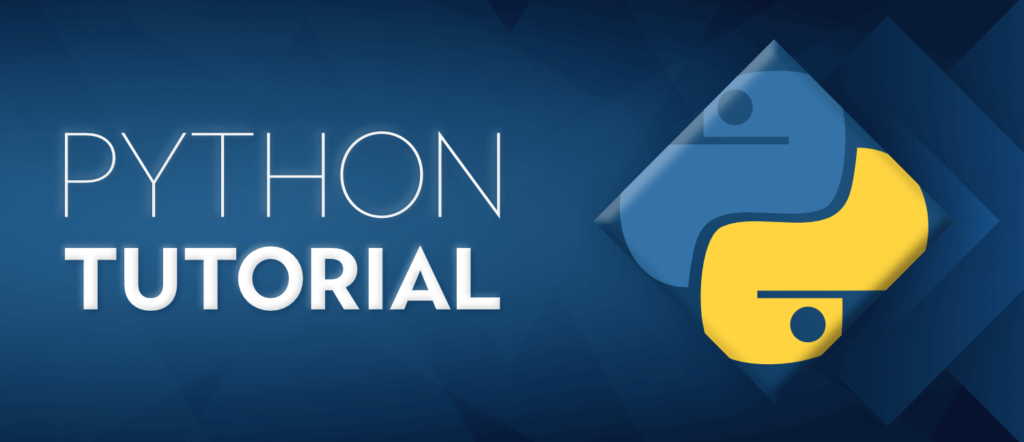
Are you interested in learning Python but don’t know where to start? You’re in the right place! Python is an excellent language for beginners, and with this guide, you’ll be coding like a pro in no time.
Why Learn Python?
If you’re considering diving into the world of programming, learning Python is a fantastic choice. This versatile language has gained immense popularity for several compelling reasons. Let’s explore why you should consider learning Python.
1. Job Prospects
Python is one of the most sought-after skills in today’s job market. Many companies across various industries are looking for developers proficient in Python. Whether you’re interested in data science, web development, artificial intelligence, or automation, Python skills can significantly enhance your employability.
- Example: Major tech companies like Google, Facebook, and Netflix rely on Python for various applications, making it a valuable skill that can open doors to exciting job opportunities.
2. Ease of Use
One of the standout features of Python is its simplicity and readability. The language is designed to be easy to learn, making it perfect for beginners. Its syntax closely resembles English, which helps new programmers grasp concepts quickly without getting bogged down by complex rules.
- Example: A beginner can write a simple program in just a few lines of code, such as printing “Hello, World!” This ease of use allows newcomers to focus on solving problems rather than struggling with complicated syntax.
print("Hello, World!")
3. Versatility
Python is incredibly versatile and can be used for a wide range of applications. From web development and data analysis to machine learning and automation, Python has libraries and frameworks that support nearly every programming need.
- Example: You can use Flask or Django for web development, Pandas for data analysis, and TensorFlow or PyTorch for machine learning projects. This versatility means that once you learn Python, you can apply your skills in various fields.
4. Strong Community Support
Python boasts a large and active community of developers who contribute to its growth and provide support for newcomers. This community creates a wealth of resources, including tutorials, forums, and libraries that make learning easier.
- Example: If you encounter challenges while coding in Python, you can find answers on platforms like Stack Overflow or join discussions in Python-related forums.
5. High Salaries
Due to the high demand for Python developers, salaries are competitive. Professionals with Python skills often command higher salaries compared to those with expertise in less popular programming languages.
- Example: According to recent salary reports, Python developers can earn an average salary that exceeds $100,000 per year in many regions, especially in tech hubs like Silicon Valley.
In summary, learning Python offers numerous benefits that can enhance your career prospects and open up new opportunities. Its ease of use makes it accessible for beginners, while its versatility allows you to work on a wide range of projects across different industries.
With strong community support and high earning potential, investing time in learning Python is a decision that can pay off significantly in your professional journey.
Take Action
- Enroll in Online Courses: Consider platforms like Coursera or Udemy to find courses tailored to beginners.
- Practice Regularly: Start working on small projects or coding challenges to reinforce what you learn.
- Join the Community: Engage with other learners and experienced developers through forums or local meetups.
Taking these steps now, you’ll be well on your way to mastering Python and unlocking a world of opportunities.
Want To Know More About:
Unlock The Secrets Of CSS: A Beginner’s Guide To Learning CSS From Scratch.
Unlock The Power Of JavaScript: A Beginner’s Guide To Learning JavaScript From Scratch
60 AI Tools To Start Your Profitable Online Business In 2025.
Setting Up Your Environment for Python Development
Before you can start coding in Python, it’s essential to set up your development environment properly. Here’s a step-by-step guide to help you get everything ready.
1. Install Python
The first step is to install Python on your computer. Follow these instructions based on your operating system:
- Windows:
- Visit the official Python website and download the latest version of Python (as of now, it’s Python 3.13.0).
- Choose the Windows installer option and run the downloaded file.
- During installation, make sure to check the boxes for “Add Python to PATH” and “Use admin privileges when installing py.exe.”
- Follow the on-screen instructions to complete the installation.
- To verify that Python is installed, open Command Prompt and type:
python -V
This command will display the installed version of Python. - macOS:
- Go to the official Python website and download the macOS installer.
- Open the downloaded
.pkg
file and follow the installation instructions. - You can verify the installation by opening Terminal and typing:
python3 -V
- Linux:
- Most Linux distributions come with Python pre-installed. However, you can install or update it using your package manager.
- For Ubuntu/Debian, open Terminal and run:
bash sudo apt-get install python3
- For Fedora, use:
bash sudo dnf install python3
- Verify the installation by typing:
bash python3 -V
2. Choose a Text Editor or IDE
Next, you’ll need a place to write your code. A text editor or Integrated Development Environment (IDE) will serve this purpose. Here are some popular choices for beginners:
- PyCharm: A powerful IDE specifically designed for Python development. It offers many features like code completion, debugging tools, and project management.
- Visual Studio Code: A lightweight yet powerful code editor that supports multiple programming languages, including Python. It has a rich ecosystem of extensions that enhance functionality.
- Sublime Text: A fast and versatile text editor that is easy to use and highly customizable.
Choose one that fits your style and start writing your first lines of code!
3. Install a Package Manager
A package manager is essential for installing and managing libraries and frameworks in Python. The most widely used package manager is pip, which comes bundled with modern versions of Python.
To check if pip is installed, open your terminal or command prompt and type:
pip --version
If it’s not installed, you can easily install it by following these steps:
- Windows: Pip is included with the Python installer from the official website.
- macOS/Linux: You can install pip using:
python3 -m ensurepip --upgrade
Once you have pip installed, you can use it to install additional libraries. For example, to install a popular library like NumPy, simply run:
pip install numpy
Setting up your environment for Python development is a straightforward process that involves installing Python, choosing a text editor or IDE, and setting up a package manager like pip. By following these steps, you’ll be well on your way to starting your coding journey in Python.
Basic Syntax and Data Types in Python
Now that you have your environment set up, it’s time to dive into some Python basics. Understanding the syntax and data types is crucial for writing effective Python code. Here are the foundational concepts you need to know:
Indentation
In Python, indentation is used to define the structure of your code, particularly for block-level constructs like loops and conditionals. Unlike many other programming languages that use braces or keywords to denote blocks of code, Python relies on consistent indentation.
- Example: Use four spaces (or a tab) to indent code blocks:
if True:
print("This is indented")
Variables
Variables in Python are used to store values. You can assign a value to a variable using the assignment operator (=
).
- Example:
x = 5 # Assigns the integer 5 to the variable x
Data Types
Python has several built-in data types that you will frequently use:
- Integers: Whole numbers without a decimal point.
x = 5 # integer
- Floats: Numbers with a decimal point.
y = 3.14 # float
- Strings: A sequence of characters enclosed in quotes.
name = "John" # string
- Lists: Ordered collections of items, which can be of different types.
fruits = ["apple", "banana", "cherry"] # list
- Dictionaries: Unordered collections of key-value pairs.
person = {"name": "John", "age": 30} # dictionary
Control Structures
Control structures are essential for controlling the flow of your program’s execution. Here are some common examples:
If/Else Statements
If/else statements allow you to execute different blocks of code based on conditions.
- Example:
x = 5
if x > 10:
print("x is greater than 10")
else:
print("x is less than or equal to 10")
For Loops
For loops are used to iterate over a sequence (like a list or string) and execute a block of code repeatedly.
- Example:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
While Loops
While loops execute a block of code repeatedly as long as a specified condition is true.
- Example:
x = 0
while x < 5:
print(x)
x += 1 # Increment x by 1
Functions
Functions are reusable blocks of code that can take arguments and return values. They help organize your code and make it more modular.
- Example:
def greet(name):
print("Hello, " + name + "!")
greet("John") # Calls the greet function with "John" as an argument
Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a programming paradigm centered around the concept of objects and classes. OOP helps organize complex programs by bundling data (attributes) and methods (functions) together.
- Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print("Hello, my name is " + self.name + " and I am " + str(self.age) + " years old.")
person = Person("John", 30) # Create an instance of Person
person.greet() # Call the greet method on the person object
Conclusion
Learning Python from scratch can seem daunting, but with this guide, you now have a solid foundation to start coding. Remember to practice regularly and experiment with different code examples to reinforce your learning.
Additional Resources
If you’re looking for more resources to help you learn Python, here are some suggestions:
- Codecademy’s Python Course: An interactive and comprehensive course that covers the basics of Python.
- Python.org: The official Python website, which has a wealth of documentation and resources for learning Python.
- Automate the Boring Stuff with Python: A free online book that teaches practical programming skills through engaging projects.
Utilizing these resources and practicing consistently, you’ll be well on your way to becoming proficient in Python.
Disclosure/Disclaimer:
This article contains affiliate links, supporting our educational and informative contents creation.
We appreciate supporting us to create more value and fascinating contents here on our site for educational purposes only.