INTRODUCTION:
Are you new to the world of web development and eager to learn the basics of CSS? Look no further! In this comprehensive guide, we’ll take you by the hand and walk you through the process of learning CSS from scratch.
What is CSS?
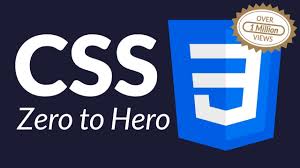
CSS stands for **Cascading Style Sheets**. It is used to define how HTML elements are displayed on screens, in print, or in other media. By using CSS, you can control various aspects of a webpage’s design, including:
Colors: Set the color of text, backgrounds, and borders.
Fonts: Choose different fonts, sizes, and styles for text.
Layouts: Arrange elements on the page using techniques like flexbox or grid.
Spacing: Control margins and padding around elements to create space.
Why Use CSS?
1. Separation of Concerns: CSS allows you to keep the content (HTML) separate from its presentation (CSS). This makes it easier to maintain and update your website’s design without altering the underlying content.
2. Consistency: By using CSS, you can ensure a consistent look across multiple pages of a website. For example, if you change the color of headings in your CSS file, that change will automatically apply to all headings throughout the site.
3. Efficiency: CSS enables you to style multiple pages at once by linking to an external stylesheet. This means you can make site-wide changes by editing just one file.
4. Responsive Design: CSS provides tools for creating responsive designs that adapt to different screen sizes and devices. This is crucial in today’s world where users access websites from various devices like smartphones, tablets, and desktops.
How Does CSS Work?
CSS works by applying styles to HTML elements through a system of selectors and rulesets:
Selectors** are patterns used to select the elements you want to style. For example:
– `h1` selects all `<h1>` elements.
– `.classname` selects all elements with a specific class.
– `#idname` selects an element with a specific ID.
Rulesets** consist of a selector followed by a set of declarations enclosed in curly braces `{}`. Each declaration includes a property and a value separated by a colon `:`. For example:
“`css
h1 {
color: blue; /* Text color */
font-size: 24px; /* Font size */
}
“`
In this example, all `<h1>` elements will be styled with blue text and a font size of 24 pixels.
Want To Learn More On:
- What Is Programming?
- Learn HTML From Scratch: HTML For Beginners – HTML Basics With Code Examples: A Step-By-Step Guide For Beginners
Types of CSS
1. **Inline CSS:** Styles are applied directly within an HTML element using the `style` attribute. This method is not recommended for large projects due to maintenance challenges.
“`html
<h1 style=”color: red;”>Hello World</h1>
“`
2. **Internal CSS:** Styles are defined within a `<style>` block in the `<head>` section of an HTML document. This method is useful for single-page styles.
“`html
<head>
<style>
h1 {
color: green;
}
</style>
</head>
“`
3. **External CSS:** Styles are defined in an external `.css` file linked to the HTML document using the `<link>` tag. This is the most efficient way for larger websites as it allows for easy updates across multiple pages.
“`html
<link rel=”stylesheet” href=”styles.css”>
CSS is an essential tool for web developers and designers, allowing for greater control over how web pages look and function. By separating content from design, CSS not only enhances user experience but also simplifies website maintenance and updates. Mastering CSS is crucial for anyone looking to create visually appealing and responsive websites that cater to users on various devices. Whether you’re building a personal blog or a professional portfolio, understanding CSS will significantly improve your web development skills.
Why Learn CSS?
CSS is an essential skill for any web developer, and learning it can open up a world of creative possibilities. With CSS, you can:
– Control the layout and appearance of web pages
– Create responsive designs that adapt to different screen sizes and devices
– Add visual effects and animations to your website
– Improve the user experience and accessibility of your website.
Basic CSS Concepts:
1. Selectors
**Selectors** are patterns used to target specific HTML elements on a web page that you want to style. They determine which elements the CSS rules will apply to. Here are some common types of selectors:
– **Element Selector:** Targets all instances of a specific HTML element. For example, `h1` selects all `<h1>` headings on the page.
“`css
h1 {
color: blue; /* This will make all <h1> elements blue */
}
“`
– **Class Selector:** Targets elements with a specific class attribute. Class selectors start with a dot (`.`). For example, `.highlight` targets all elements with the class “highlight”.
“`css
.highlight {
background-color: yellow; /* This will highlight elements with the class “highlight” */
}
“`
– **ID Selector:** Targets an element with a specific ID attribute. ID selectors start with a hash (`#`). For example, `#header` targets the element with the ID “header”.
“`css
#header {
font-size: 24px; /* This will set the font size of the element with ID “header” */
}
“`
2. Properties:
**Properties** define the aspects of an element that you want to style. Each property corresponds to a specific style attribute in CSS. Here are some commonly used properties:
– **Color:** Sets the color of text or borders.
“`css
p {
color: green; /* This will make all <p> text green */
}
“`
– **Background-color:** Sets the background color of an element.
“`css
div {
background-color: lightblue; /* This will set the background color of all <div> elements to light blue */
}
“`
– **Font-size:** Defines the size of text.
“`css
h2 {
font-size: 20px; /* This will set all <h2> text to be 20 pixels in size */
}
“`
3. Values:
**Values** specify what you want for each property. Values can be various data types, such as colors, sizes, lengths, or keywords. Here are examples of different types of values:
– **Color Values:** Can be specified using names (like `red`), hexadecimal codes (like `#FF0000`), or RGB values (like `rgb(255,0,0)`).
– **Size Values:** Can be specified in pixels (e.g., `16px`), ems (e.g., `1em`), percentages (e.g., `100%`), or viewport units (e.g., `vw`, `vh`).
### Example of a CSS Ruleset
Putting it all together, here’s how a simple CSS ruleset looks:
“`css
h1 {
color: blue; /* Property: color | Value: blue */
font-size: 24px; /* Property: font-size | Value: 24 pixels */
text-align: center; /* Property: text-align | Value: center */
}
“`
In this example:
– The selector is `h1`, targeting all `<h1>` elements.
– The properties are `color`, `font-size`, and `text-align`.
– The corresponding values are `blue`, `24px`, and `center`.
Understanding these basic CSS concepts—selectors, properties, and values—is crucial for anyone looking to style web pages effectively. By mastering these elements, you can create visually appealing designs and layouts that enhance user experience across different devices and screen sizes. As you progress in your CSS journey, these foundational concepts will serve as building blocks for more advanced techniques and styling methods.
Practical Examples:
Now that we have a solid understanding of CSS concepts, let’s put that knowledge into practice with some practical examples. These examples will demonstrate how to style different HTML elements using CSS.
Example 1: Create a Heading Element with a Level of 1 and Set Its Color to Red
To create a heading (an `<h1>` element) and set its color to red, you can use the following CSS:
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Heading Example</title>
<style>
h1 {
color: red; /* This sets the text color of all <h1> elements to red */
}
</style>
</head>
<body>
<h1>This is a Red Heading</h1>
</body>
</html>
“`
Example 2: Create a Paragraph Element and Set Its Background Color to Blue
To create a paragraph (`<p>`) element and set its background color to blue, you can use the following CSS:
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Paragraph Example</title>
<style>
p {
background-color: blue; /* This sets the background color of all <p> elements to blue */
color: white; /* Optional: Sets the text color to white for better contrast */
padding: 10px; /* Optional: Adds some padding around the text */
}
</style>
</head>
<body>
<p>This is a paragraph with a blue background.</p>
</body>
</html>
“`
Example 3: Create a Link Element and Set Its Font Size to 18 Pixels
To create a link (`<a>`) element and set its font size to 18 pixels, you can use the following CSS:
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Link Example</title>
<style>
a {
font-size: 18px; /* This sets the font size of all <a> elements to 18 pixels */
color: blue; /* Sets the link color to blue */
text-decoration: none; /* Optional: Removes the underline from links */
}
a:hover {
text-decoration: underline; /* Optional: Underlines the link on hover for better interaction feedback */
}
</style>
</head>
<body>
<a href=”#”>This is a link with 18px font size.</a>
</body>
</html>
“`
Summary of Examples:
– **Example 1** demonstrates how to style an `<h1>` heading by changing its color to red.
– **Example 2** showcases how to give a `<p>` paragraph a blue background while also enhancing its readability with padding and contrasting text color.
– **Example 3** illustrates how to change the font size of an `<a>` link and enhance user experience by modifying its appearance on hover.
These examples highlight how CSS can be used effectively to enhance the visual presentation of HTML elements. By practicing these styles, you’ll gain confidence in your ability to create attractive and functional web pages.
Conclusion:
Learning CSS from scratch can seem daunting, but with practice and patience, you can master the basics of CSS. Remember to start with simple selectors, properties, and values, and gradually build up to more complex styles. With CSS, the possibilities are endless, and we hope this guide has inspired you to start creating your own amazing web designs.
Disclosure/Disclaimer:
This article contains affiliate links, supporting our educational and informative contents creation.
We appreciate supporting us to create more value and fascinating contents here on our site for educational purposes only.